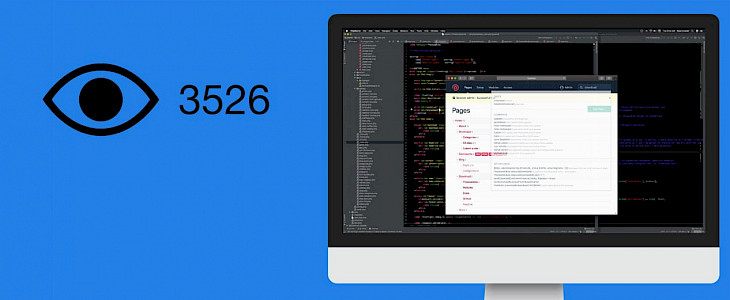
Count Views Module for ProcessWire allows you to save page views directly to database, and display them when required. This free module can be used to count views of post or count file downloads in ProcessWire. The module offers two public functions ->show and ->add.
To count views of post in ProcessWire you can for example create a field "count" with type "Integer" and set it as "hidden". Then you can define the count field to selected template, and start to count page views using the code below.
$page->count += 1;
$page->of(false);
$page->save('count');
$page->of(true);
echo $page->count; // to display actual value
The downside is, that the "count" filed will change the modification date of the page.
For some reasons you can be not interested in updating page modification date, and then ProcessWire CountViews Module will come in handy. For example you like to change the modification date only when the content will change, and not when it's viewed by user. Let's see how the ProcessWire CountViews Module PHP code looks like in action.
<?php
class PageViews extends WireData implements Module
{
public static function getModuleInfo()
{
return array(
'title' => __('Count Views'),
'summary' => __('Count page views.'),
'version' => '100',
'author' => 'Kuba Pawlak',
'autoload' => true
);
}
const table = 'page_views';
public function init()
{
$this->addHookProperty('Page::views', $this, 'hookGetViewsForPage');
$this->pages->addHookAfter('save', $this, 'afterPageSave');
}
public function ___install()
{
$table = self::table;
$this->database->exec("CREATE TABLE IF NOT EXISTS `$table` (
`page_id` int(11) NOT NULL,
`views` int(11) NOT NULL,
PRIMARY KEY (`page_id`),
KEY `page_id` (`views`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1 ;");
}
public function ___uninstall()
{
$table = self::table;
$this->database->exec("DROP TABLE `$table`;");
}
public function hookGetViewsForPage(HookEvent $event)
{
$page = $event->object;
$event->return = PageViewsAccessor::initWithPage($page);
}
}
class PageViewsAccessor
{
protected $page;
protected static $instances = array();
public function __construct(Page $page)
{
$this->page = $page;
self::$instances[$page->id] = $this;
}
public static function initWithPage(Page $page)
{
if (isset(self::$instances[$page->id])) return self::$instances[$page->id];
return new self($page);
}
public function __get($i)
{
if (method_exists($this, $i)) {
return $this->$i();
}
return null;
}
public function show()
{
$table = PageViews::table;
$query = wire('database')->prepare("SELECT views from $table where page_id = ? ");
$query->execute(array($this->page->id));
if(!$query->rowCount()) return null;
$id = $query->fetchColumn();
echo (int) $id;
}
public function add()
{
$table = PageViews::table;
$query = wire('database')->prepare("SELECT views from $table where page_id = ? ");
$query->execute(array($this->page->id));
if(!$query->rowCount()) {
$statement = wire('database')->prepare("INSERT INTO $table (page_id, views) VALUES (?,?)");
$statement->execute(array($this->page->id, '1'));
}
else {
$id = $query->fetchColumn();
$dwn = (int) $id + 1;
$statement = wire('database')->prepare("UPDATE $table SET views = ? WHERE page_id = ?;");
$statement->execute(array($dwn, $this->page->id));
}
}
}
How to use ProcessWire Count Views Module?
After you install CountViews Module you can easily access ->add and ->view options. The functions work in every ProcessWire template, and to start use the code available below.
$page->views->add(); // add +1 to views on actual page
$id = 2456;
$pages->get($id)->views->add() // add +1 to views to page $id
$page->views->show(); // displays actual view value for page
$id = 3456;
$pages->get($id)->views->show() // display view value for page with $id